- Blog/
How to setup Redis cache for Gin Web Framework
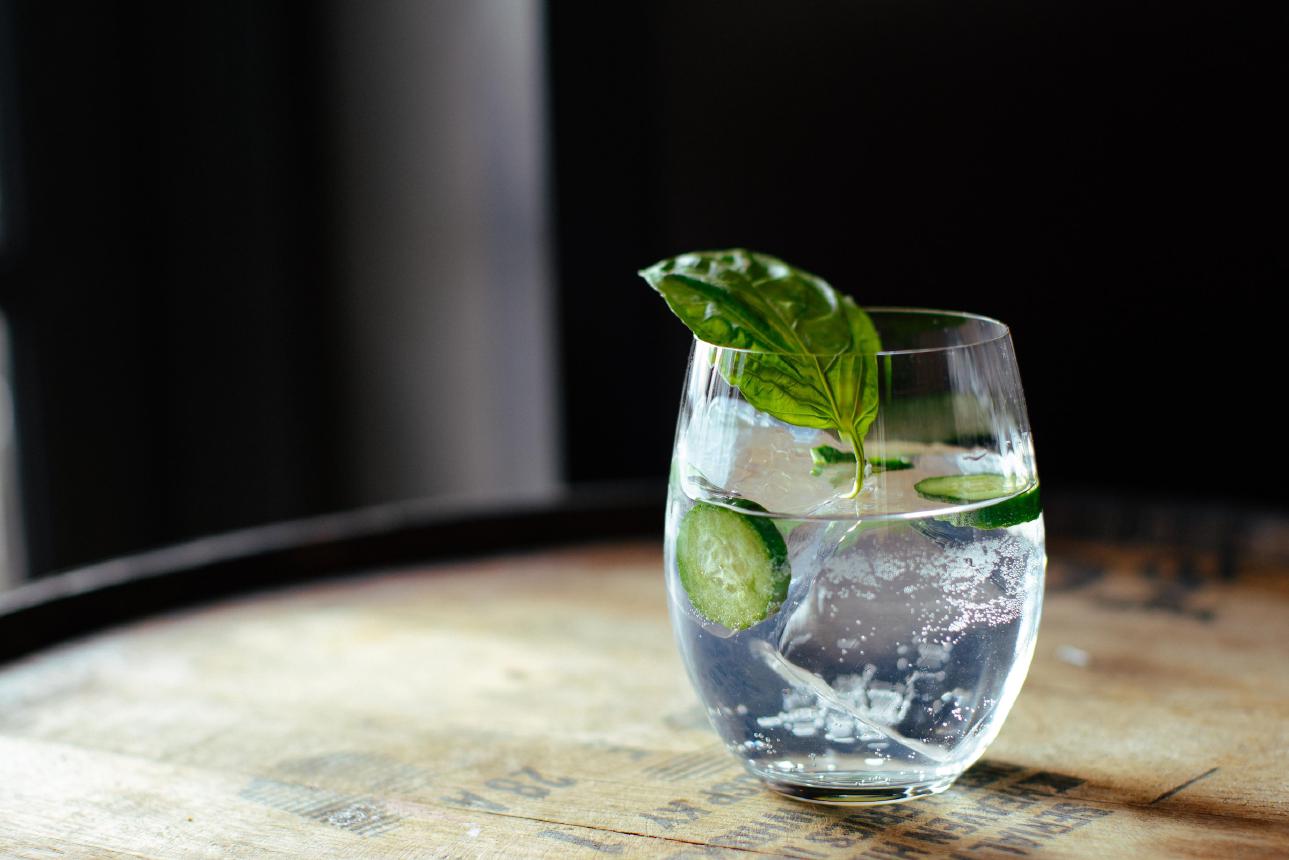
Table of Contents
Introduction #
When we access a website, we anticipate a swift loading experience. If it lags, we tend to navigate elsewhere. Hence, the speed of websites is crucial. Employing a cache is a key method to boost website speed. A cache serves as a brief repository for swiftly accessible data. While many websites utilize CDNs (Content Delivery Networks) to cache static content such as images, CSS, and JavaScript files, what about dynamic content?
Certain queries exhibit varying response times due to disparities in processing power and database access requirements. For queries that don’t necessitate real-time data, we can implement caching to expedite their results.
In this post, we’ll explore the process of configuring Redis caching for Go Gin Tonic, allowing you to optimize your API queries by retrieving cached responses without accessing the database.
Prerequisites #
What is Gin Framework #
Gin is a popular web framework for building web applications and APIs in the Go programming language (Golang). It’s designed to be minimalistic yet highly efficient, making it a top choice for developers who want to create performant applications with minimal boilerplate code.
The key features of the Gin web framework for Go (Golang) in brief are:
- High Performance: Known for its speed and efficiency.
- Lightweight: Minimal overhead, with flexibility to add components.
- Middleware Support: Easily integrate functionality like authentication and logging.
- Efficient Routing: Intuitive routing system for URL handling.
- Validation: Built-in support for data validation.
- Error Handling: Effective error management.
- JSON Parsing: Simplified JSON handling.
- Modular: Use as a standalone framework or integrate with other libraries.
What is Redis #
Redis, short for “Remote Dictionary Server,” is an open-source, in-memory data store and caching system. It is often referred to as a “data structure server” because it supports various data structures like strings, lists, sets, hashes, and more. Redis is known for its exceptional speed and is widely used as a caching mechanism and real-time data store.
Getting Started #
1. Install Gin Framework #
Let’s start by installing Gin Framework. To do so, run the following command:
$ go get -u github.com/gin-gonic/gin
2. Create a Go file #
First, create a file called example.go:
# assume the following codes in main.go file
$ touch main.go
3. Let’s create a basic Gin application #
Create a basic Gin application in the main.go file:
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
4. Create Redis Cache configuration #
package main
import (
"fmt"
"os"
"time"
"github.com/counterapi/api/pkg"
"github.com/chenyahui/gin-cache/persist"
"github.com/go-redis/redis/v8"
)
// RedisCache is a config struct for redis cache.
type RedisCache struct {
Store *persist.RedisStore
DefaultCacheTime time.Duration
}
// SetupRedisCache sets the redis up.
func SetupRedisCache() *RedisCache {
return &RedisCache{
Store: persist.NewRedisStore(redis.NewClient(&redis.Options{
Network: "tcp",
Addr: fmt.Sprintf(
"%s:%s",
os.Getenv("REDIS_HOST"),
os.Getenv("REDIS_PORT"),
),
})),
DefaultCacheTime: 10 * time.Second,
}
}
5. Let’s use the config to cache the response #
Use the RedisCache
struct to cache the response of /ping
endpoint. This will cache the response for 10 seconds.
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
cacheConfig := SetupRedisCache()
r.GET(
"/ping",
cache.CacheByRequestURI(cacheConfig.Store, cacheConfig.DefaultCacheTime),
func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
Conclusion #
To boost website speed, one of the most effective techniques is the use of caching. Caching involves temporarily storing frequently accessed data so that it can be rapidly retrieved without the need to access the original data source. While Content Delivery Networks (CDNs) are commonly used to cache static assets like images and scripts, the question remains: how can we efficiently cache dynamic content?
This blog post has explored the process of setting up Redis caching for Go Gin Tonic, a popular web framework for building high-performance web applications and APIs in the Go programming language (Golang). By leveraging Redis, you can optimize your API queries by retrieving cached responses, eliminating the need for repeated database access.
By following these steps, you can harness the power of Redis caching to enhance the speed and responsiveness of your Go Gin Tonic applications, ensuring a smoother and more efficient user experience. Caching dynamic content has never been easier, thanks to the combination of Gin and Redis.